I only needed one of the fields, which contained a date string. Using arcMap, I was able to solve this by using the field calculator to extract it. If you only need to extract a couple fields, this may work for you, albeit a bit tedious:
The following steps will help you build up a line of VB to use in the field calculator to cut a sub-string from the description field based on the HTML tags and the legth of data stored in your table.
- In GoogleEarth, open your KML/KMZ, click on a feature to open the popup. Identify the exact name of the field you want to retrive. Mine is called "DATE"
- In ArcMap, open the attribute table of the layer that you created when imported the KML/KMZ.
- Identify the description field. In my data it is called "PopupInfo"
- In the first record, right-click in the "PopupInfo" cell, and click "copy"
- Open Notepad and paste the contents of the cell. You will now see the HTML for the pop-up.
- Use ctrl-F to find the field name from the KML/KMZ (in my case, "DATE"). Here is a screenshot showing just the line of the HTML I care about.
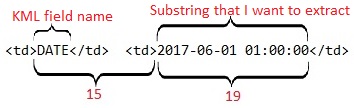
- Count the number of characters, including spaces from the beginning of the field name until the start of your data. In my case there are 15 characters.
- Determine how many characters you want to extract. For my substring, I need 19. For example if you just need state codes like AZ and WY, you may only need 2. If your data doesn't have consistent length entries in this field, err on the side of the longest. Edit: you can remove the extra characters later manually or by using the instr() and rtrim() VB functions. Post or message me if you need help with that.
In arcMap, add a new TEXT field your layer, and be sure to give it at least as many characters as you'll need for your data. The default is 50.
Then, open the field calculator for your new field. The start of the data is found using the InStr() function, and the data is extracted using the Mid() function. Type the following VB command:
Mid([PopupInfo],InStr([PopupInfo],"DATE")+15,19)
Of course, swap out my field names for yours (from steps 3 and 6), and change the length of the numbers 15 and 19 to the length of your substrings (found in steps 7 and 8).
In the VB line above:
- [PopupInfo] is the name of the annoying field that contains the HTML
for the KML pop-up.
- "DATE" is the KML field name and HTML tag that contains data that I want to extract.
- 15 is the number of characters from the start of the date tag to the start of the actual date data.
- 19 is the number of characters in thee date string that we want to
retain.