For now, I can only suggest Python solutions that can be turned into a custom function via the Function Editor.
So, there is a polygon layer called 'polygon', which was the last modified on: 2024-12-03 09:31:33
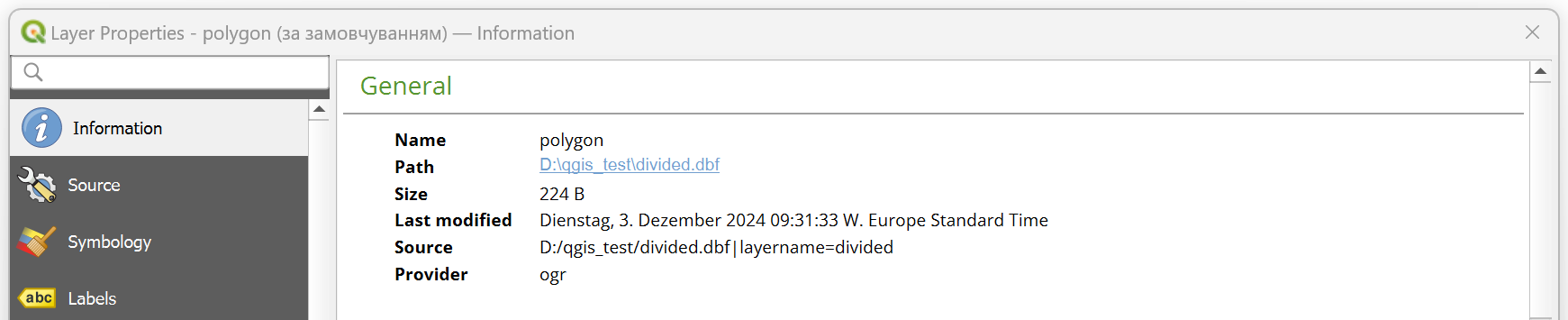
There are several of them:
Through the getmtime()
function from the os.path
Python module:
Return the time of last modification of path. The return value is a
floating-point number giving the number of seconds since the epoch
(see the
time
module). Raise
OSError
if the file does not exist or is inaccessible.
# imports
from os.path import isfile, getmtime
from datetime import datetime
from qgis.core import QgsProject
# getting the vector layer by its name
layer = QgsProject.instance().mapLayersByName("polygon")[0]
# getting the file path of the data source
source_path = layer.dataProvider().dataSourceUri().split('|')[0]
# checking if the source is a file and get its last modified time
if isfile(source_path):
# getting the last modified time using getmtime
modified_time = getmtime(source_path)
# formating the timestamp for readability
formatted_time = datetime.fromtimestamp(modified_time).strftime('%A, %d. %B %Y %H:%M:%S')
print(f"Last modified: {formatted_time}")
else:
print("The layer source is not a file or its modification date cannot be determined.")
Utilizing the st_mtime()
function from the os
Python module:
Get the status of a file or a file descriptor. Perform the equivalent
of a stat()
system call on the given path. path may be specified as
either a string or bytes – directly or indirectly through the
PathLike
interface – or as an open file descriptor. Return a
stat_result
object.
# imports
from os import stat
from os.path import isfile
from datetime import datetime
from qgis.core import QgsProject
# getting the vector layer by its name
layer = QgsProject.instance().mapLayersByName("polygon")[0]
# getting the file path of the data source
source_path = layer.dataProvider().dataSourceUri().split('|')[0]
# checking if the source is a file and get its last modified time
if isfile(source_path):
# using os.stat to get file metadata
file_stats = stat(source_path)
# getting the last modified time using st_mtime
modified_time = file_stats.st_mtime
# formating the timestamp for readability
formatted_time = datetime.fromtimestamp(modified_time).strftime('%A, %d. %B %Y %H:%M:%S')
print(f"Last modified: {formatted_time}")
else:
print("The layer source is not a file or its modification date cannot be determined.")
The output is: Last modified: Tuesday, 03. December 2024 09:31:33
As @J.R mentioned in their comment, information about the last modification could be data-type dependent, see for example Vector Drivers for more details. So, when one uses the ogr
module, it could provide you with the DBF_DATE_LAST_UPDATE
parameter for a shapefile:
DBF_DATE_LAST_UPDATE=YYYY-MM-DD
: Modification date to write in DBF
header with year-month-day format. If not specified, current date is
used.
# imports
from qgis.core import QgsProject
from datetime import datetime
from osgeo import ogr
# getting the vector layer by its name
layer = QgsProject.instance().mapLayersByName("polygon")[0]
# getting the file path of the data source
source_path = layer.dataProvider().dataSourceUri().split('|')[0]
# openning the vector layer with ogr module
dataSource = ogr.Open(source_path)
daLayer = dataSource.GetLayer(0)
# getting the last modified time
modified_time = daLayer.GetMetadata_Dict().get('DBF_DATE_LAST_UPDATE')
print(f"Last modified: {modified_time}")
The output is: Last modified: 2024-12-03
.
now()
as default value, and force update that field upon feature creation or edit.