Probably not as good as the approach offered by @MrXsquared, however, it does not require scripting and purely based on domestic tools&functions.
Let's assume we have five features in 'poly_test' (purplish) and ten in 'points' (orange) accordingly, see image below. As you can see there once in a while several point per same location.
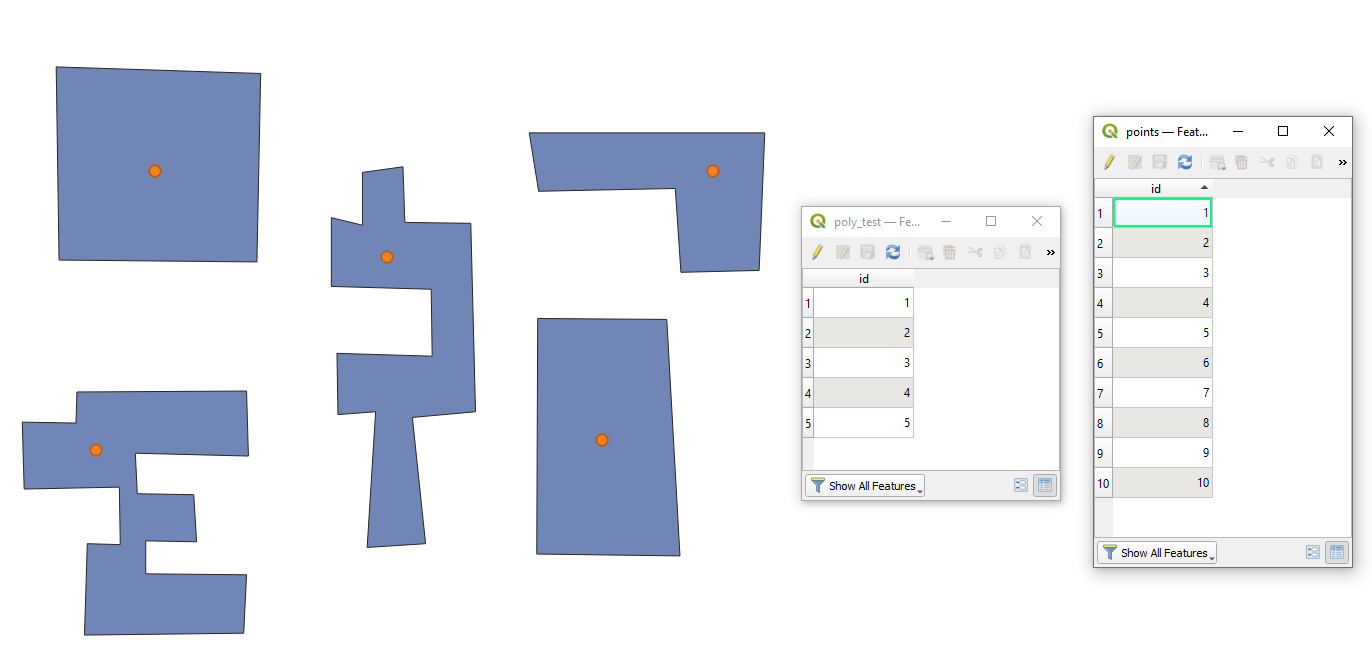
Here are several steps that can be executed to achieve the desired output.
Step 1. Count points in polygon and concatenate a unique attribute of those points. Alternatively the "Count points in polygon" can be used.
In the Field calculator it can be done via array_length(overlay_contains('points', expression:="id"))
for number of points and array_to_string(overlay_contains('points', expression:="id"))
for concatenation of unique attribute
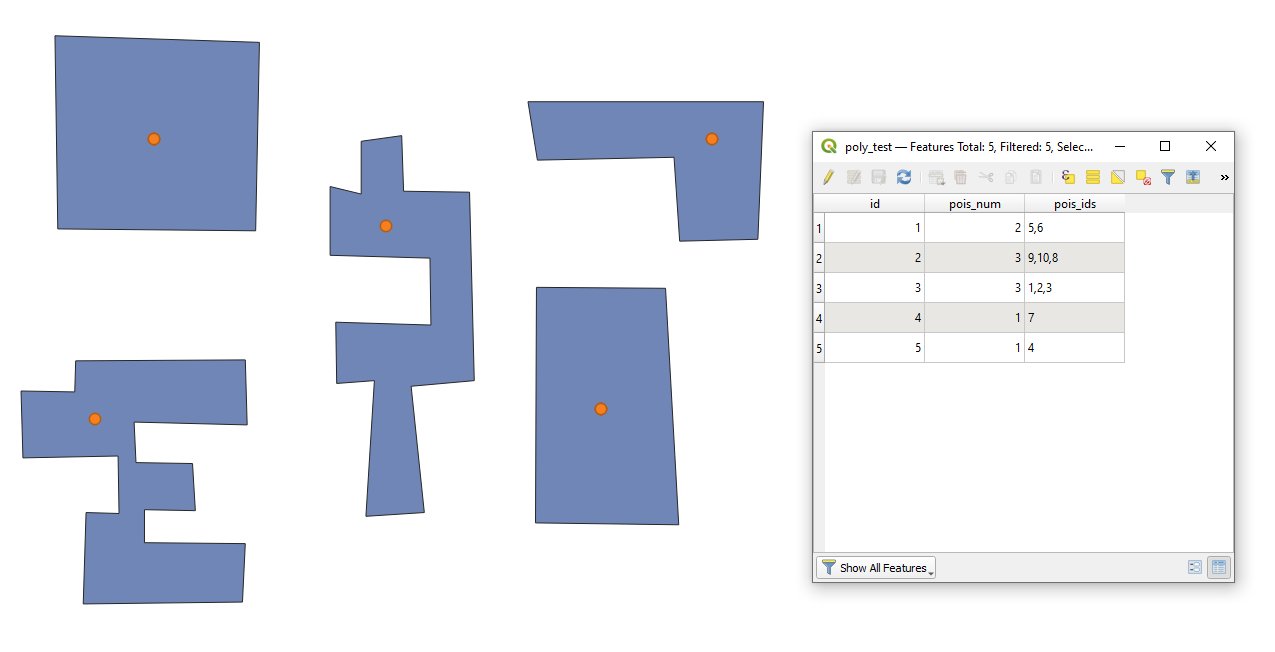
Step 2. Run the "Random points inside polygons" geolgorithm with specifying the "pois_num"
field as one for 'Point count or density' (I used Points count for 'Sampling strategy')
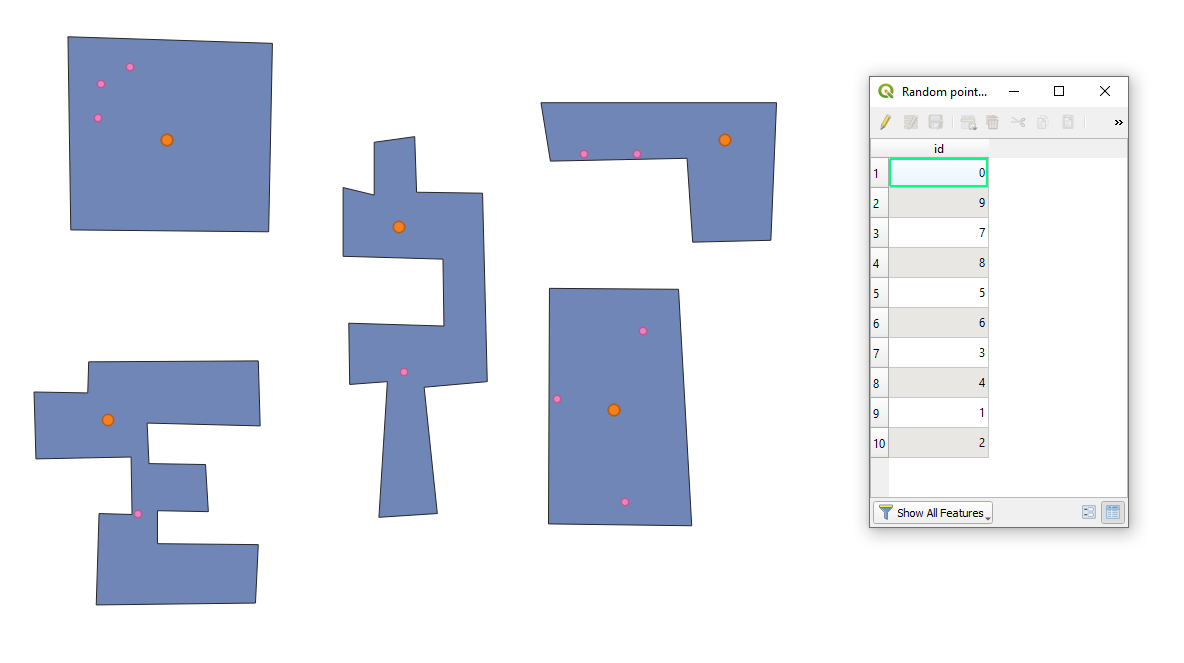
If needed filter split polygons into ones with only one point and ones that contain more than one point.
Step 3. Apply the "Join attributes by location" to get attributes of polygons
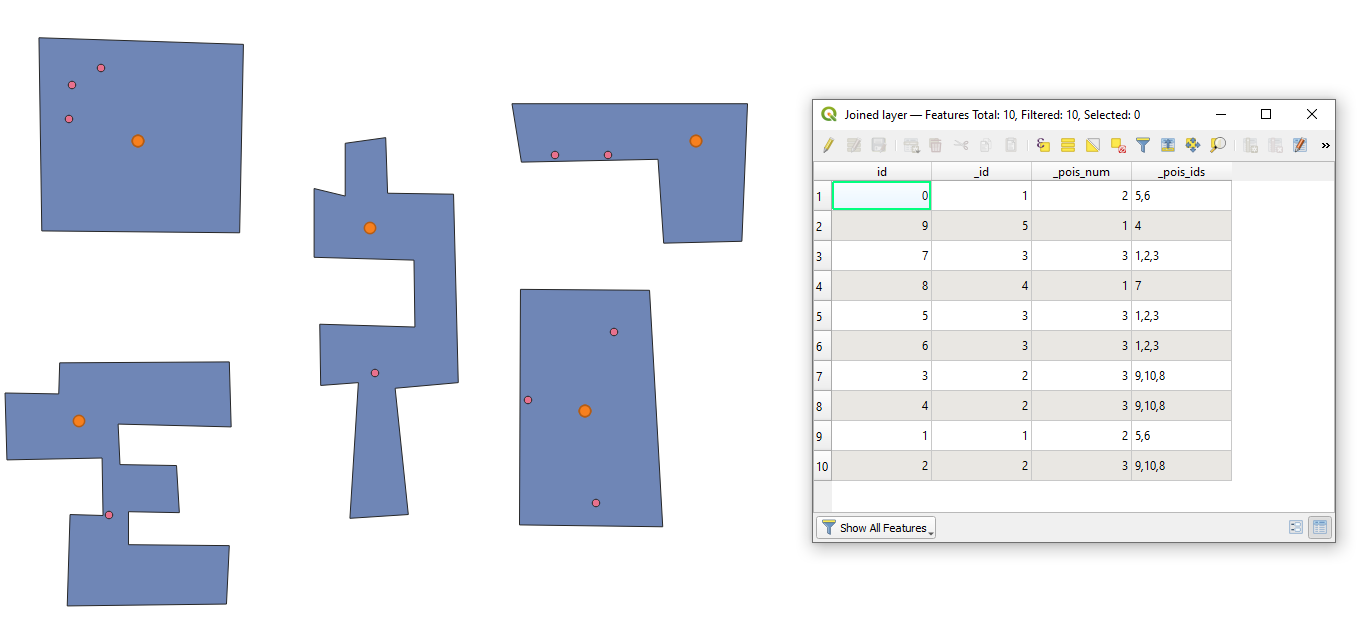
Step 4. "Add autoincremental field" to make a new "id"
i.e. index within each group (a set of points and a polygon feature). Polygons' "_id"
was used for 'Group values by [optional]'
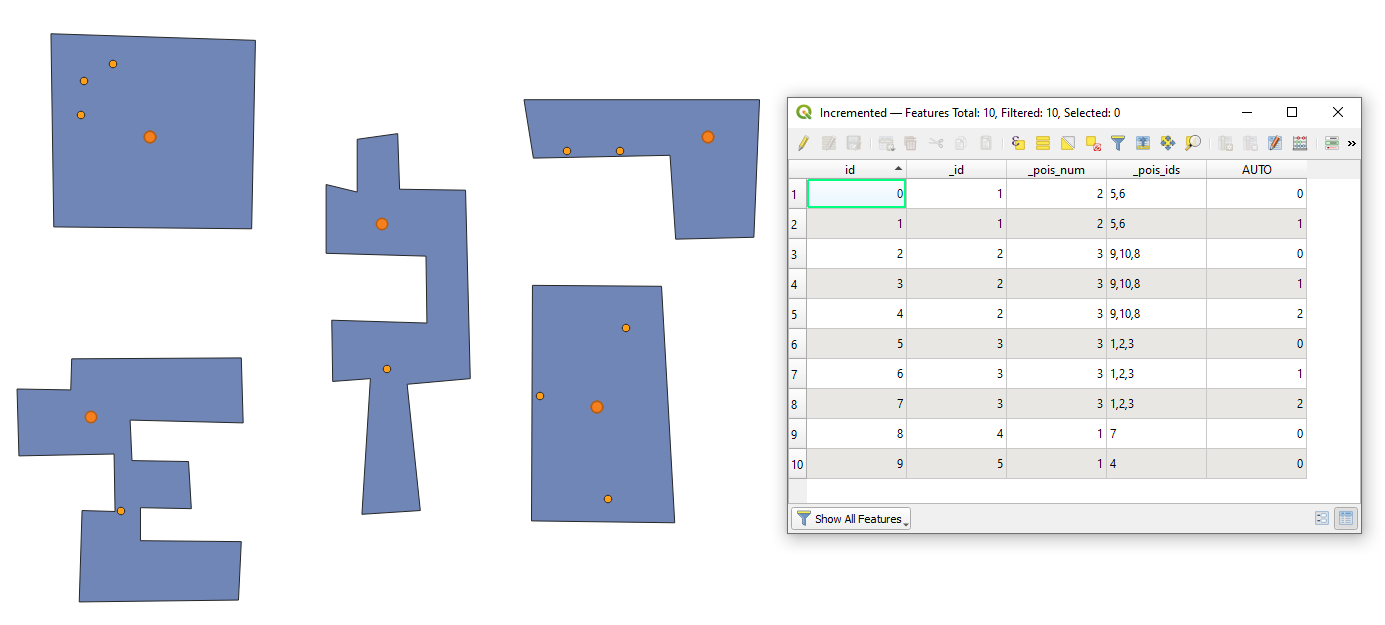
Step 5. Get back the "id"
s of the original point layer using the array_get(string_to_array("_pois_ids"),"AUTO")
in the Field Calculator. Other attributes from the original point layer can achieved further via a joining to the "id"
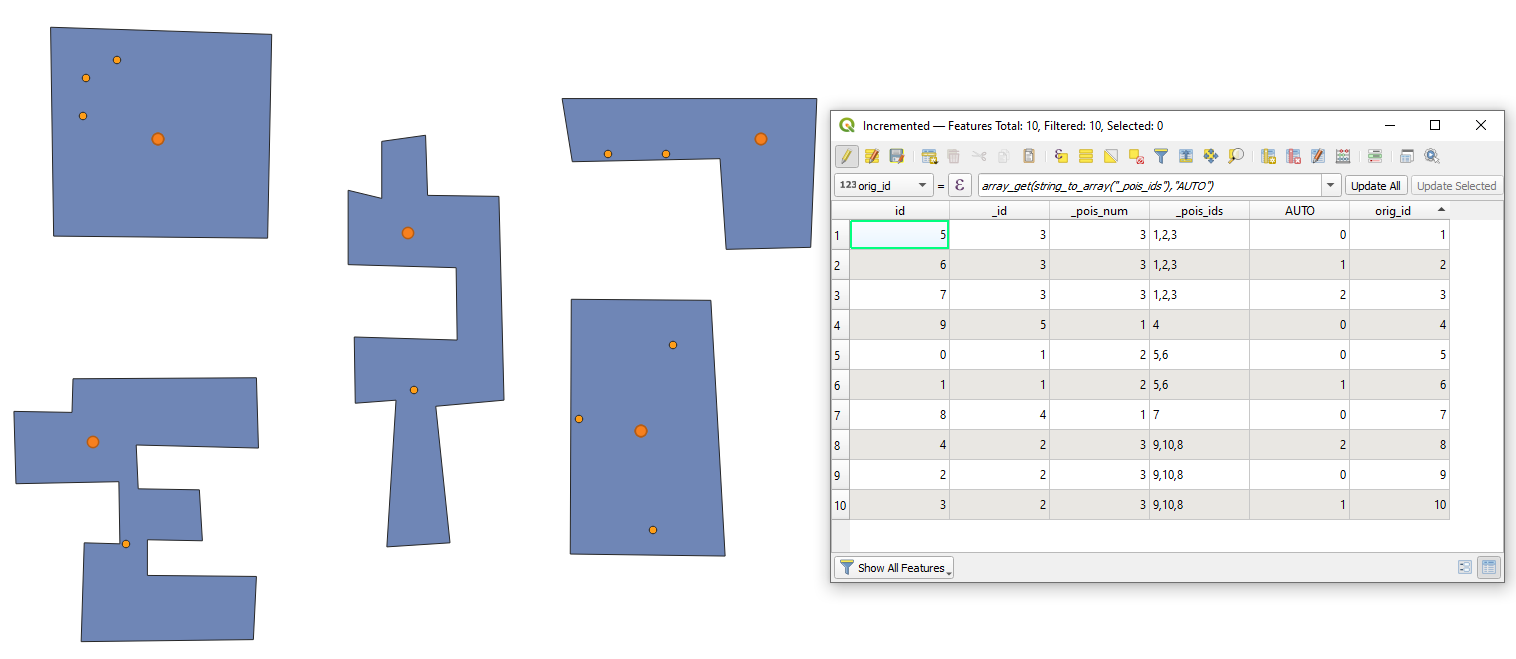
P.S. If the procedure must be repeated multiple times then the above workflow recommended to transform into a model
I also tried a "Virtual Layer" through Layer > Add Layer > Add/Edit Virtual Layer...
with the following query
SELECT poly.id AS id1,
poi.id AS id2,
point_on_surface(poly.geometry) AS geom
FROM "poly_test" AS poly
CROSS JOIN "points" AS poi ON st_within(poi.geometry, poly.geometry)
Unfortunately it does not create a new point on surface for each point feature. The same will be expected using the "Point on surface" geoalgorithm.
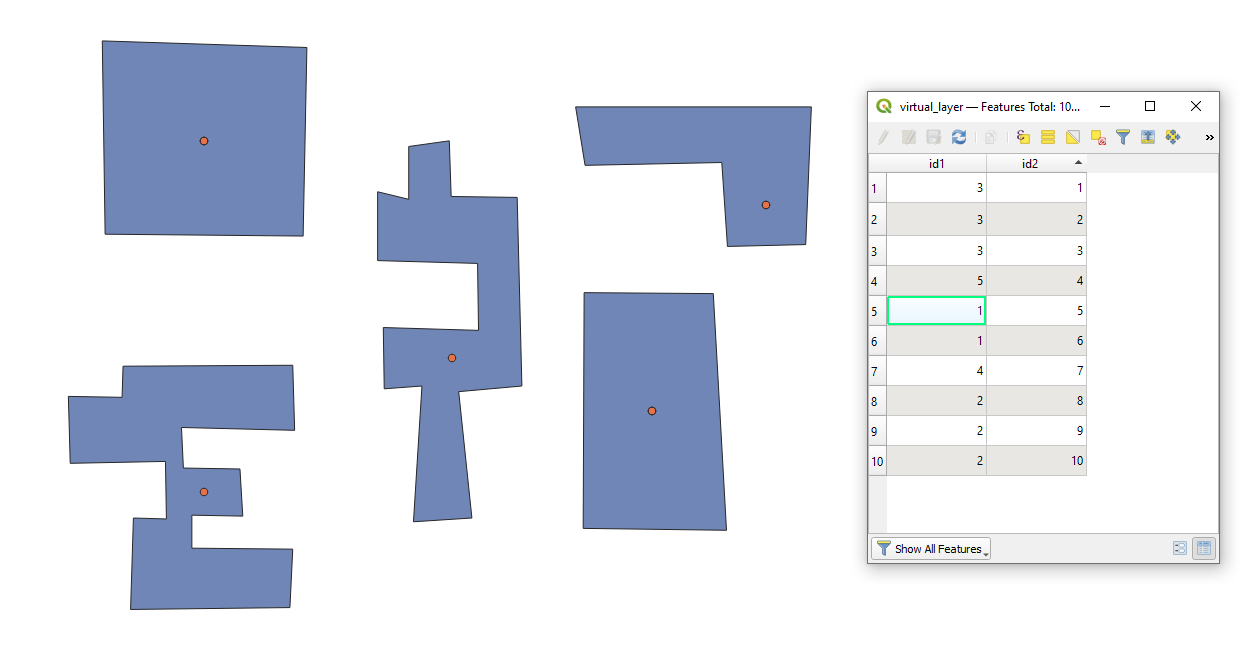