Since you said you get a group of intersecting polygons for each polygon you're interested in, you may want to create what is referred to as a "polygon overlay".
This isn't exactly what Adam's solution is doing. To see the difference, take a look at this picture of an ABC intersection:
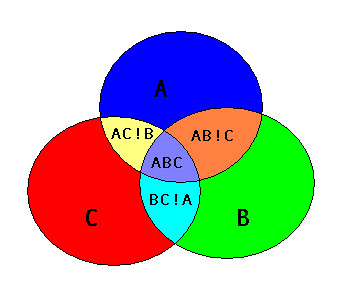
I believe Adam's solution will create an "AB" polygon that covers both the area of "AB!C" and "ABC", as well as an "AC" polygon that covers "AC!B" and "ABC", and a "BC" polygon that is "BC!A" and "ABC". So the "AB", "AC", and "BC" output polygons would all overlap the "ABC" area.
A polygon overlay produces non-overlapping polygons, so AB!C would be one polygon and ABC would be one polygon.
Creating a polygon overlay in PostGIS is actually pretty straightforward.
There are basically three steps.
Step 1 is extract the linework [Note that I'm using the exterior ring of the polygon, it does get a little more complicated if you want to correctly handle holes]:
SELECT ST_ExteriorRing(polygon_col) AS the_geom FROM my_table) AS lines
Step 2 is to "node" the linework (produce a node at every intersection). Some libraries like JTS have "Noder" classes you can use to do this, but in PostGIS the ST_Union function does it for you:
SELECT ST_Union(the_geom) AS the_geom FROM (...your lines...) AS noded_lines
Step 3 is to create all the possible non-overlapping polygons that can come from all those lines, done by the ST_Polygonize function:
SELECT ST_Polygonize(the_geom) AS the_geom FROM (...your noded lines...)
You could save the output of each of those steps into a temp table, or you can combine them all into a single statement:
CREATE TABLE my_poly_overlay AS
SELECT geom FROM ST_Dump((
SELECT ST_Polygonize(the_geom) AS the_geom FROM (
SELECT ST_Union(the_geom) AS the_geom FROM (
SELECT ST_ExteriorRing(polygon_col) AS the_geom FROM my_table) AS lines
) AS noded_lines
)
)
I'm using ST_Dump because the output of ST_Polygonize is a geometry collection, and it is (usually) more convenient to have a table where each row is one of the polygons that makes up the polygon overlay.