I created a Python (2.7) script quite a while back where I ported formulas over to Python. I don't claim to understand all the math behind it but I do know it does work.
If your coordinates are in a geographic coordinate system (e.g. 41.977880,-91.665623) this script could work or you could at least reverse engineer the code's math to fit your needs.
The formula information for calculating bearings was taken from this page under the "Bearing" topic.
This code was part of my answer for this question on Getting all vertex lat, long coordinates every 1 meter between two known points using PHP?. So there is also a port of this code to PHP as well as Javascript.
Here is my python code to get the azmuth in degrees:
import math
def calculateBearing(lat1,lng1,lat2,lng2):
'''calculates the azimuth in degrees from start point to end point'''
startLat = math.radians(lat1)
startLong = math.radians(lng1)
endLat = math.radians(lat2)
endLong = math.radians(lng2)
dLong = endLong - startLong
dPhi = math.log(math.tan(endLat/2.0+math.pi/4.0)/math.tan(startLat/2.0+math.pi/4.0))
if abs(dLong) > math.pi:
if dLong > 0.0:
dLong = -(2.0 * math.pi - dLong)
else:
dLong = (2.0 * math.pi + dLong)
bearing = (math.degrees(math.atan2(dLong, dPhi)) + 360.0) % 360.0;
return bearing
#Cedar Rapids, Iowa
startLatitude = 41.977880
startLongitude = -91.665623
#Chicago, IL
endLatitude = 41.878114
endLongitude = -87.629798
azimuth = calculateBearing(startLatitude ,startLongitude ,endLatitude ,endLongitude)
print "Your Initial Azmuth Would be {0} Degrees".format(round(azimuth,2))
The output of this script would look like the following:

Hope this helps or at least gets you on the right track.
Also, you could just plug that function into that field calculator if you have the correct input and it could calculate the azimuth without major modification with the field calculator like so:
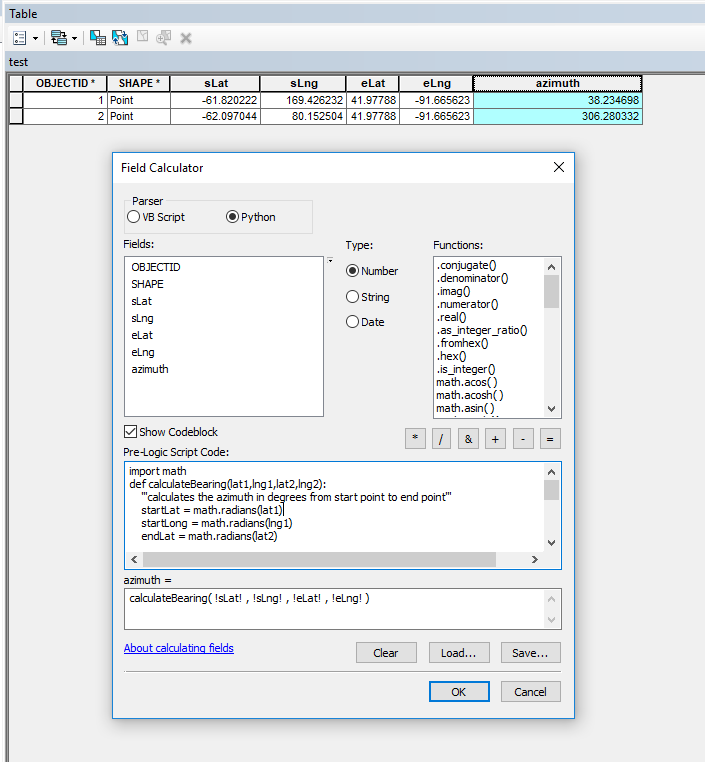