There are several things regarding your code:
the uri
for a CSV file must include the file://
prefix, as it is mentioned in the QGIS Documentation:
The provider string is structured as a URL, so the path must be prefixed with file://
.
there is a method called isValid()
from the QgsDataProvider
class:
Returns true if this is a valid layer. It is up to individual providers to determine what constitutes a valid layer.
As was already mentioned by @BERA in his comment the reason why you do not see anything on your map canvas is because your coordinates are with ,
(562834,932) instead of .
(562834.932).
And there are several solutions available:
A solution without changing the input CSV file
This solution based on adding the decimalPoint=','
info the uri
.
#Set up inputs
absolute_path_to_csv_file = '/C:/Users/taras/Downloads/POINTS_.csv'
encoding = 'UTF-8'
delimiter = ';'
decimal = ','
crs = 'epsg:32632'
x = 'UTM32_E'
y = 'UTM32_N'
uri = f"file://{absolute_path_to_csv_file}?encoding={encoding}&delimiter={delimiter}&decimalPoint={decimal}&crs={crs}&xField={x}&yField={y}"
#Make a vector layer
layer = QgsVectorLayer(uri, "Points", "delimitedtext")
#Check if layer is valid
if not layer.isValid():
print ("Layer not loaded")
#Add CSV data
QgsProject.instance().addMapLayer(layer)
A solution with modifying the input CSV file
There are several solutions available for replacing all commas with dots in your input CSV file:
- Notepad
- some Python (This solution maybe better covered on the StackOverflow)
absolute_path_to_files = 'C:/Users/taras/Downloads/'
input = open(absolute_path_to_files + 'POINTS.csv', "r")
text = ''.join([i for i in input]).replace(',','.')
output = open(absolute_path_to_files + 'POINTS_.csv', "w")
output.writelines(text)
output.close()
And then use the following code (either refer to an updated CSV file or a newly created)
#Set up inputs
absolute_path_to_csv_file = '/C:/Users/taras/Downloads/POINTS_.csv'
encoding = 'UTF-8'
delimiter = ';'
crs = 'epsg:32632'
x = 'UTM32_E'
y = 'UTM32_N'
uri = f"file://{absolute_path_to_csv_file}?encoding={encoding}&delimiter={delimiter}&crs={crs}&xField={x}&yField={y}"
#Make a vector layer
layer = QgsVectorLayer(uri, "Points", "delimitedtext")
#Check if layer is valid
if not layer.isValid():
print ("Layer not loaded")
#Add CSV data
QgsProject.instance().addMapLayer(layer)
to get the output like this:
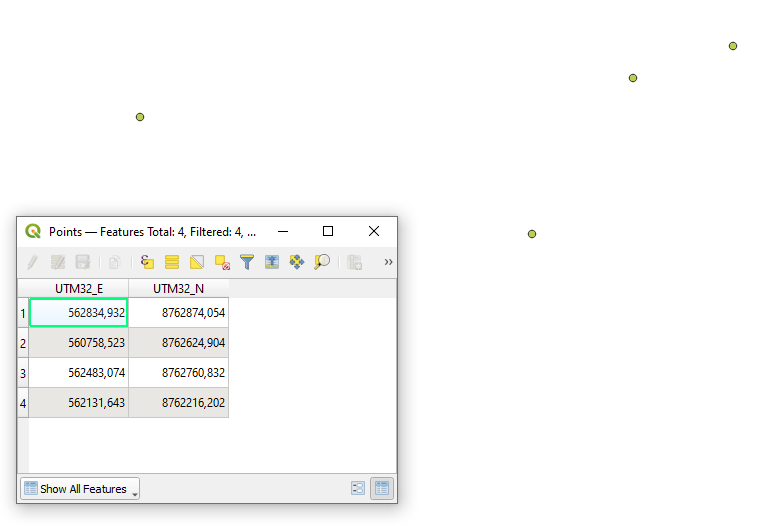
References:
562834,932
to562834.932
and so on